티스토리 뷰
클래스 구성
** 기능 클래스 / ~ 데이터용 클래스
** MainActivity
** StudyActivity
** EditActivity
~Voca
~Storage
"팝업 - 커스텀다이얼로그"
- 팝업창의 레이아웃을 xml파일로 inflate하여 임의로 지정이 가능하다.
- StudyActivity에서 SWAP2 버튼을 클릭하면 RadioButton이 뜬다.
이때 RadioButton은 꼭 RadioGroup안에 묶어주어야 중복선택을 방지할 수 있다.
RadioGroup에서 RadioButton들을 나열하는 방법은 android:orientation로 지정가능하다.
'Inflater'
}else if(view.getId() == R.id.swap_bt2){
//인플레이터
LayoutInflater Inf = (LayoutInflater) getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View v = Inf.inflate(R.layout.activity_custom_dialog, null, false); //팝업창 레이아웃 미리 짜둔 거
//라디오버튼 중복 체크 안되도록 radiogroup으로 xml에서 묶어줘야 함
RadioButton korRb = v.findViewById(R.id.kor_r);
final RadioButton engRb = v.findViewById(R.id.eng_r);
engRb.setChecked(isEng);
//다이얼로그
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("한영모드 전환");
builder.setView(v);
builder.setNegativeButton("취소", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int i) {
}
});
builder.setPositiveButton("확인", new DialogInterface.OnClickListener(){
@Override
public void onClick(DialogInterface dialog, int i) {
if(isEng != engRb.isChecked()){
isEng = engRb.isChecked();
init();
}
}
});
builder.create().show();
}
}
"INTENT(화면전환)"
-StudyActivity에서 화면전환 버튼을 클릭하면 OptionActivity 페이지로 넘어가게되고
Back을 누르면 다시 되돌아온다.
-여기서도 역시 한/영 모드 설정이 가능하다.
StudyActivity에서의 코드
}else if(view.getId()==R.id.swap_bt3){ //인텐트로 화면전환
Intent intent = new Intent(this, com.example.ex_voca.OptionActivity.class);
intent.putExtra("isEng", isEng);
startActivityForResult(intent, 1000);
OptionActivity에서의 코드
boolean isEng = getIntent().getBooleanExtra("isEng", false); //플래그
engRb.setChecked(isEng);
findViewById(R.id.btn).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int result = engRb.isChecked() ? 0 : -1; //이건 짧게 줄인 버전
더보기
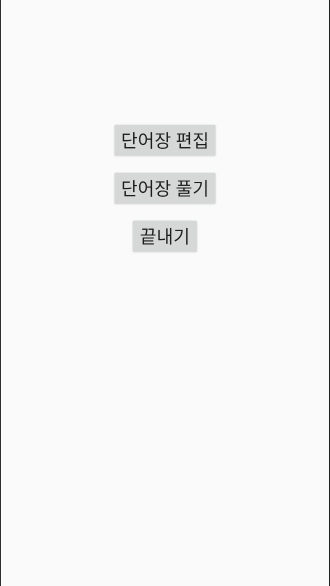
전체코드 보기
MainActivity
package com.example.ex_voca;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
Button btEdit;
Button btStudy;
Button btQuit;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
btEdit = findViewById(R.id.bt_edit);
btStudy = findViewById(R.id.bt_study);
btQuit = findViewById(R.id.bt_quit);
Storage.vocaArr.add(new Voca("dog","개"));
Storage.vocaArr.add(new Voca("cat","고양이"));
Storage.vocaArr.add(new Voca("candy","캔디"));
Storage.vocaArr.add(new Voca("carmel","낙타"));
Storage.vocaArr.add(new Voca("car","차"));
btEdit.setOnClickListener(this);
btStudy.setOnClickListener(this);
btQuit.setOnClickListener(this);
}
@Override
public void onClick(View view) {
if (view.getId() == R.id.bt_edit) {
// Intent intent = new Intent(this, my.real.voca.EditActivity.class);
// startActivity(intent);
startActivity(new Intent(this, com.example.ex_voca.EditActivity.class));
} else if (view.getId() == R.id.bt_study) {
startActivity(new Intent(this, com.example.ex_voca.StudyActivity.class));
} else if (view.getId() == R.id.bt_quit) {
finish();
}
}
}
activity_main.xml
img
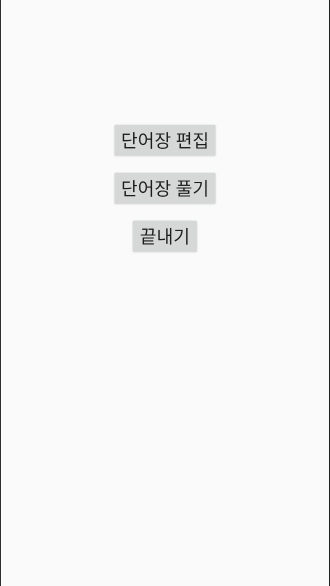
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<Button
android:id="@+id/bt_edit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_marginTop="150dp"
android:text="단어장 편집"
android:textSize="23sp" />
<Button
android:id="@+id/bt_study"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/bt_edit"
android:layout_centerHorizontal="true"
android:layout_marginTop="10dp"
android:text="단어장 풀기"
android:textSize="23sp" />
<Button
android:id="@+id/bt_quit"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/bt_study"
android:layout_centerHorizontal="true"
android:layout_marginTop="10dp"
android:text="끝내기"
android:textSize="23sp" />
</RelativeLayout>
EditActivity : 단어수정
package com.example.ex_voca;
import androidx.appcompat.app.AlertDialog;
import androidx.appcompat.app.AppCompatActivity;
import android.content.DialogInterface;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
import java.util.ArrayList;
import java.util.Random;
public class EditActivity extends AppCompatActivity implements View.OnClickListener, AdapterView.OnItemClickListener {
EditText engEt;
EditText korEt;
Button addBt;
ListView contentLv;
int idx;
boolean isEdit = false;
ArrayList<String> arr = new ArrayList<>();
ArrayAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_edit);
engEt = findViewById(R.id.eng_et);
korEt = findViewById(R.id.kor_et);
addBt = findViewById(R.id.add_bt);
contentLv = findViewById(R.id.content_lv);
adapter = new ArrayAdapter(this, android.R.layout.simple_list_item_1, arr);
contentLv.setAdapter(adapter);
show();
addBt.setOnClickListener(this);
contentLv.setOnItemClickListener(this);
}
private void show(){
arr.clear();
for (int i = 0; i < Storage.vocaArr.size(); i++) {
Voca v = Storage.vocaArr.get(i);
arr.add(v.eng + " : "+v.kor);
}
adapter.notifyDataSetChanged();
}
@Override
public void onClick(View view) {
if(isEdit){
String eng = engEt.getText().toString().trim();
String kor = korEt.getText().toString().trim();
engEt.setText("");
korEt.setText("");
Storage.vocaArr.get(idx).eng = eng;
Storage.vocaArr.get(idx).kor = kor;
// Voca voca = new Voca(eng, kor);
// Storage.vocaArr.set(idx, voca);
isEdit =false;
}else {
String eng = engEt.getText().toString();
String kor = korEt.getText().toString();
Voca v = new Voca(eng, kor);
Storage.vocaArr.add(v);
engEt.setText("");
korEt.setText("");
}
show();
}
@Override
public void onItemClick(AdapterView<?> adapterView, View view, final int idx, long l) {
this.idx = idx;
final Voca temp = Storage.vocaArr.get(idx);
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("수정 또는 삭제 할래?");
builder.setMessage(temp.eng + " : " +temp.kor);
builder.setPositiveButton("삭제", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
Storage.vocaArr.remove(idx);
show();
}
});
builder.setNegativeButton("수정", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialogInterface, int i) {
engEt.setText(temp.eng);
korEt.setText(temp.kor);
addBt.setText("수정");
isEdit = true;
}
});
builder.setCancelable(true);
builder.create().show();
}
}
activity_edit.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".EditActivity">
<Button
android:id="@+id/add_bt"
android:layout_width="80dp"
android:layout_height="wrap_content"
android:layout_alignTop="@+id/eng_et"
android:layout_alignBottom="@+id/kor_et"
android:layout_alignParentRight="true"
android:layout_marginRight="15dp"
android:text="입력"
android:textSize="23sp" />
<EditText
android:id="@+id/eng_et"
android:layout_width="match_parent"
android:layout_height="50dp"
android:layout_margin="15dp"
android:layout_toLeftOf="@+id/add_bt"
android:hint="영어"
android:paddingLeft="5dp" />
<EditText
android:id="@+id/kor_et"
android:layout_width="match_parent"
android:layout_height="50dp"
android:layout_below="@+id/eng_et"
android:layout_margin="15dp"
android:layout_toLeftOf="@+id/add_bt"
android:hint="한글"
android:paddingLeft="5dp" />
<ListView
android:id="@+id/content_lv"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_below="@+id/add_bt"
android:layout_alignLeft="@+id/eng_et"
android:layout_alignRight="@+id/add_bt"
android:layout_marginTop="15dp"
android:layout_marginBottom="15dp"
android:background="#dfdfdf"
android:padding="10dp"
android:textSize="20sp" />
</RelativeLayout>
StudyActivity : 단어게임 클래스
package com.example.ex_voca;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import android.app.AlertDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ProgressBar;
import android.widget.RadioButton;
import android.widget.RelativeLayout;
import android.widget.TextView;
import android.widget.Toast;
public class StudyActivity extends AppCompatActivity implements View.OnClickListener {
int idx;
int score;
boolean isEng;
/** 알림 글 **/
TextView noticeTv;
/** 실행 화면 **/
TextView scoreTv;
TextView countTv;
TextView idxTv;
TextView questionTv;
EditText answerEt;
Button swapBt;
Button swapBt2;
Button swapBt3;
Button submitBt;
ProgressBar idxBar;
RelativeLayout questionLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_study);
idxBar = findViewById(R.id.idx_bar);
noticeTv = findViewById(R.id.notice_tv);
scoreTv = findViewById(R.id.score_tv);
idxTv = findViewById(R.id.idx_tv);
questionTv = findViewById(R.id.question_tv);
answerEt = findViewById(R.id.answer_et);
swapBt = findViewById(R.id.swap_bt);
swapBt2 = findViewById(R.id.swap_bt2);
swapBt3 = findViewById(R.id.swap_bt3);
submitBt = findViewById(R.id.submit_bt);
countTv = findViewById(R.id.count_tv);
questionLayout = findViewById(R.id.question_layout);
isEng = true;
if(Storage.vocaArr.size() < 1){
noticeTv.setVisibility(View.VISIBLE);
questionLayout.setVisibility(View.INVISIBLE);
}else{
init();
noticeTv.setVisibility(View.INVISIBLE);
questionLayout.setVisibility(View.VISIBLE);
idxBar.setMax(Storage.vocaArr.size());
submitBt.setOnClickListener(this);
swapBt.setOnClickListener(this);
swapBt2.setOnClickListener(this); // 이거 안해주니까 안눌리지ㅠㅠㅠㅠㅠㅠㅠㅠ
swapBt3.setOnClickListener(this);
time =5;
countTv.setText(String.valueOf(time));
handler.sendEmptyMessageDelayed(0, 1000);
}
}
private void init(){
idx = 0;
score =0;
showQuestion();
}
int time = 5;
Handler handler = new Handler(){
@Override
public void handleMessage(@NonNull Message msg) {
super.handleMessage(msg);
time--;
if(time > 0){
countTv.setText(String.valueOf(time));
handler.sendEmptyMessageDelayed(0, 1000);
}else{
nextQuestion();
}
}
};
private void showQuestion(){
Voca voca = Storage.vocaArr.get(idx);
if(isEng){
questionTv.setText(voca.eng);
}else{
questionTv.setText(voca.kor);
}
scoreTv.setText(score+"점");
idxTv.setText((idx+1)+"/"+Storage.vocaArr.size());
idxBar.setProgress(idx+1);
}
private void nextQuestion(){
idx++;
//10 -> 0-9
if(idx == Storage.vocaArr.size()){
//모든 문제 풀었음
scoreTv.setText(score+"점");
Toast.makeText(this, "완료!", Toast.LENGTH_SHORT).show();
}else{
showQuestion();
time = 100;
countTv.setText(String.valueOf(time));
handler.removeMessages(0);
handler.sendEmptyMessageDelayed(0, 1000);
}
}
@Override
protected void onDestroy() {
super.onDestroy();
handler.removeMessages(0);
}
@Override
public void onClick(View view) {
if(view.getId() == R.id.submit_bt){
Voca voca = Storage.vocaArr.get(idx);
String input = answerEt.getText().toString().trim();
answerEt.setText("");
String answer = "";
answer = isEng ? voca.kor : voca.eng;
// if(isEng){
// answer = voca.kor;
// }else{
// answer = voca.eng;
// }
if(input.equals(answer)){
score += 20;
}else{
//오답
}
nextQuestion();
}else if(view.getId() == R.id.swap_bt ) {
isEng = !isEng; //요기 있는 이 스왑이 밑에 줄 setChecked에서 확인되는지
init();
}else if(view.getId()==R.id.swap_bt3){ //인텐트로 화면전환
Intent intent = new Intent(this, com.example.ex_voca.OptionActivity.class);
intent.putExtra("isEng", isEng);
startActivityForResult(intent, 1000);
}else if(view.getId() == R.id.swap_bt2){
//인플레이터
LayoutInflater Inf = (LayoutInflater) getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View v = Inf.inflate(R.layout.activity_custom_dialog, null, false); //팝업창 레이아웃 미리 짜둔 거
//라디오버튼 중복 체크 안되도록 radiogroup으로 xml에서 묶어줘야 함
RadioButton korRb = v.findViewById(R.id.kor_r);
final RadioButton engRb = v.findViewById(R.id.eng_r);
engRb.setChecked(isEng);
//다이얼로그
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("한영모드 전환");
builder.setView(v);
builder.setNegativeButton("취소", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int i) {
}
});
builder.setPositiveButton("확인", new DialogInterface.OnClickListener(){
@Override
public void onClick(DialogInterface dialog, int i) {
if(isEng != engRb.isChecked()){
isEng = engRb.isChecked();
init();
}
}
});
builder.create().show();
}
}
}
activity_study.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".StudyActivity">
<TextView
android:id="@+id/notice_tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:layout_margin="20dp"
android:text="저장된 문제가 없습니다"
android:textSize="30sp"
android:visibility="gone" />
<RelativeLayout
android:id="@+id/question_layout"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ProgressBar
android:id="@+id/idx_bar"
android:layout_width="match_parent"
android:max="100"
android:progress="10"
style="@android:style/Widget.Material.ProgressBar.Horizontal"
android:layout_height="wrap_content"
android:layout_alignTop="@+id/score_tv"
android:layout_centerHorizontal="true"
android:layout_toLeftOf="@+id/idx_tv"
android:layout_toRightOf="@+id/score_tv" />
<TextView
android:id="@+id/score_tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:text="20점"
android:textSize="30sp" />
<TextView
android:id="@+id/count_tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="20dp"
android:layout_below="@+id/score_tv"
android:text="5"
android:textSize="30sp" />
<TextView
android:id="@+id/idx_tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_margin="20dp"
android:text="1/10"
android:textSize="30sp" />
<Button
android:id="@+id/swap_bt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/idx_tv"
android:layout_alignParentRight="true"
android:layout_margin="20dp"
android:text="swap"
android:textSize="30sp" />
<Button
android:id="@+id/swap_bt2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/swap_bt"
android:layout_alignParentRight="true"
android:layout_margin="20dp"
android:text="swap2"
android:textSize="30sp" />
<Button
android:id="@+id/swap_bt3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/swap_bt2"
android:layout_alignParentRight="true"
android:layout_margin="20dp"
android:text="화면전환"
android:textSize="30sp" />
<TextView
android:id="@+id/question_tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:layout_margin="20dp"
android:text="Dog"
android:textSize="30sp" />
<EditText
android:id="@+id/answer_et"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/question_tv"
android:layout_centerHorizontal="true"
android:layout_margin="10dp"
android:hint="입력"
android:minWidth="150dp"
android:textSize="30sp" />
<Button
android:id="@+id/submit_bt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/answer_et"
android:layout_centerHorizontal="true"
android:layout_margin="10dp"
android:hint="확인"
android:minWidth="150dp"
android:textSize="30sp" />
</RelativeLayout>
</RelativeLayout>
Voca
package com.example.ex_voca;
public class Voca {
String eng;
String kor;
public Voca(String eng, String kor) {
this.eng = eng;
this.kor = kor;
}
}
Storage
package com.example.ex_voca;
import java.util.ArrayList;
public class Storage {
public static ArrayList<Voca> vocaArr = new ArrayList<>();
}
'TEMP' 카테고리의 다른 글
비전공자 취준 넋두리 & 관련 링크 모음 (0) | 2021.03.01 |
---|---|
방향키로 공 움직이기+총 쏘기_옵저버패턴, AWT, class, method (0) | 2020.09.04 |
캔버스에 색 넣기(Canvas 상속) (0) | 2020.09.03 |
Sep1_멀티스레드에서 우선순위 지정(flag 개념) (0) | 2020.09.01 |
Sep1_멀티쓰레드 (0) | 2020.09.01 |
댓글